In the world of object-oriented programming (OOP), the concept of a root or base class is foundational for understanding class inheritance and the relationships between different classes.
In this post, we’ll dive deep into the role of the root class in Swift and its relationship with NSObject
within the context of Objective-C and Cocoa
frameworks.
Whether you’re building an app in Swift or working with legacy Objective-C code, grasping the role of the root class is key to designing robust, scalable software.
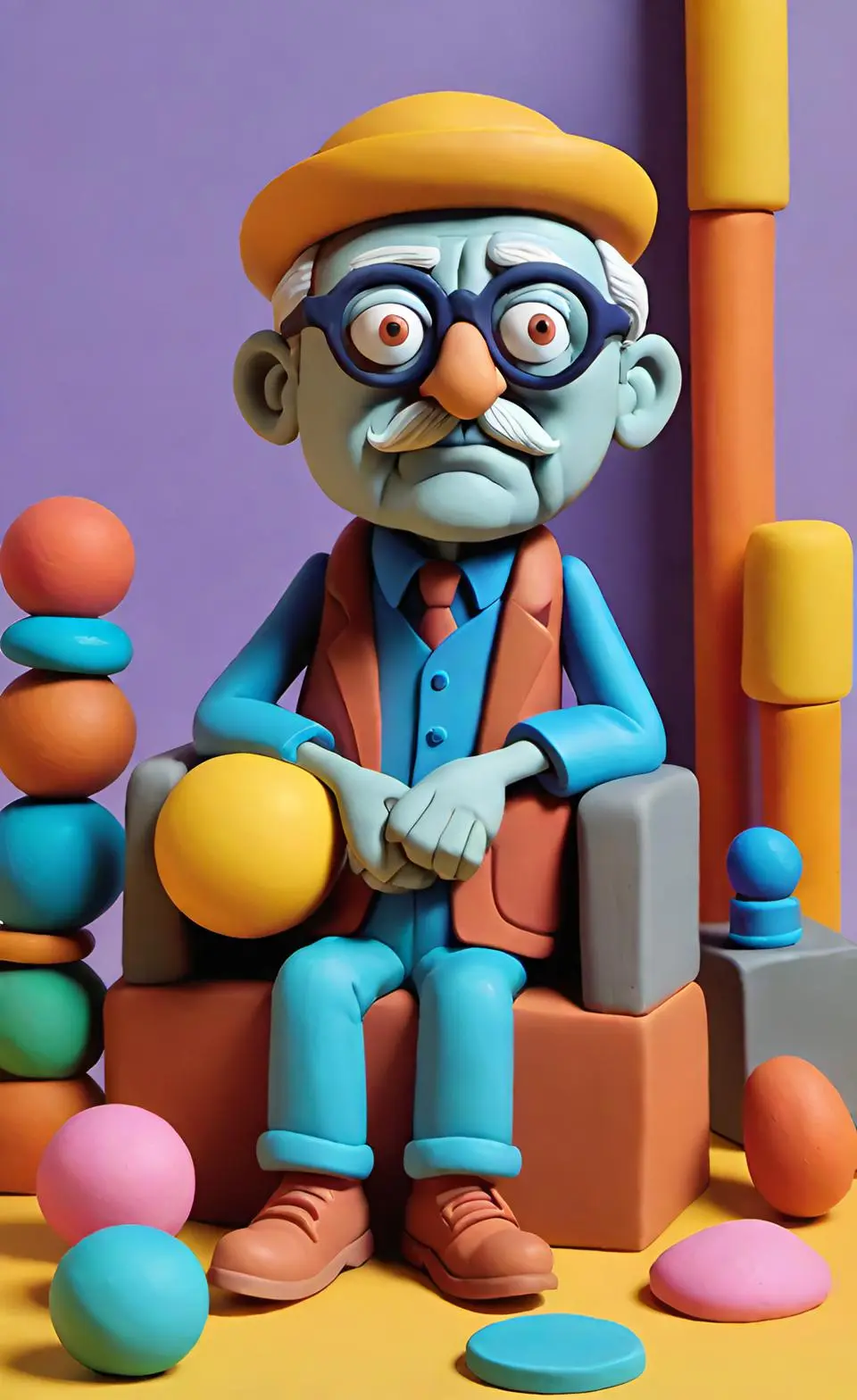
Understanding the Concept of a Root Class
In object-oriented programming, a root class, also known as a base class, is the topmost class in an inheritance hierarchy. It acts as the foundation upon which all other classes are built. Essentially, all subclasses inherit the properties and methods of the root class, making it a critical element in the design of any object-oriented system.
Key Characteristics of a Root Class:
- Inheritance: A root class provides default implementations for properties and methods that can be inherited and potentially overridden by subclasses.
- Polymorphism: The root class enables polymorphic behavior, allowing subclasses to be treated as instances of the root class, enabling flexible and reusable code.
- Common Interface: It often defines a common interface that all subclasses adhere to, ensuring consistency across the object hierarchy.
Root Class in Objective-C and Cocoa
In Objective-C the de facto root class for nearly all objects within the Cocoa
and Cocoa Touch
frameworks is NSObject
, a part of the Foundation
framework. This means that virtually every class in these frameworks ultimately derives from NSObject
, either directly or indirectly.
NSObject in Detail
NSObject
is more than just a root class; it’s the cornerstone of the entire Cocoa
framework. It provides a wealth of essential methods that support basic object behavior, including:
- Memory Management: With methods like
retain
,release
, andautorelease
,NSObject
plays a pivotal role in manual memory management under the older retain/release model, and it’s still relevant in the context of ARC (Automatic Reference Counting). - Method Swizzling:
NSObject
facilitates powerful runtime features like method swizzling, allowing developers to dynamically change method implementations at runtime. - Equality and Hashing:
NSObject
defines fundamental methods such asisEqual:
andhash
, which are crucial for comparing objects and storing them in collections likeNSSet
orNSDictionary
. - KVC & KVO: It also supports Key-Value Coding (KVC) and Key-Value Observing (KVO), allowing properties to be accessed and monitored dynamically at runtime.
Root Class in Swift
In Swift, when you define a class without specifying a superclass, it automatically becomes a root class, reflecting a deliberate design choice aimed at simplicity and safety. This approach is similar to Objective-C, where although NSObject
is commonly used as the root class, it is not strictly enforced. Developers can create classes that do not inherit from any other class, allowing for custom root classes. For instance, Apple’s API includes multiple root classes, such as NSObject
, NSProxy
, and the now-deprecated Object.
The historical reason for having a root class, particularly in Objective-C, was to ensure that all objects shared a common interface, including essential methods like isEqualTo:
, hash()
, and others required for collection classes. However, with the advent of generics in Swift, the necessity of a root class has diminished.
In Swift, many of the methods traditionally provided by NSObject
have been distributed across protocols such as Equatable
, Hashable
, and CustomStringConvertible
, which allows both classes and structs to share common interfaces. This approach enhances Swift’s flexibility and aligns with its protocol-oriented nature.
Although a universal root class is not necessary in pure Swift, you can still inherit from NSObject
when working with Objective-C APIs, ensuring smooth interoperability. It’s also important to understand that Swift classes do not run on top of Objective-C; they are compiled by the same compiler, which allows for seamless interoperation between Swift and Objective-C when needed. This is why the @objc
attribute is sometimes required to ensure consistency with Objective-C protocols and classes.
Swift’s Flexibility and Interoperability
Swift does not have a universal base class that all classes inherit from by default. However, it is still designed to interoperate seamlessly with Objective-C, allowing developers to leverage existing Cocoa
frameworks. This interoperability is facilitated through:
- @objc Attribute: This attribute marks Swift classes, methods, and properties to be compatible with Objective-C, enabling them to be exposed to and used by Objective-C code. This ensures that Swift and Objective-C can coexist within the same project, allowing for mixed-language development.
- NSObject: While not required, Swift classes can inherit from
NSObject
to gain access to Objective-C runtime features. This is particularly useful when working with Cocoa APIs that rely on dynamic features such as KVO (Key-Value Observing) or when a class needs to be compatible with Objective-C’s expectations, such as when it needs to be part of an Objective-C collection or needs to respond to selectors.
Practical Implications
When working within a mixed Swift/Objective-C codebase, understanding when and why to subclass NSObject
is critical. For example:
- Interfacing with Cocoa Frameworks: If your Swift class needs to interact with
Cocoa
frameworks that rely on Objective-C runtime features, subclassingNSObject
may be necessary. - Using KVO: If you plan to use Key-Value Observing, your Swift class must inherit from
NSObject
because KVO is deeply tied to the Objective-C runtime.
Conclusion
Understanding the role of root classes is essential for developers working with object-oriented languages like Swift and Objective-C. In Objective-C, NSObject
serves as a commonly used root class, providing a rich set of functionalities that underpin the Cocoa
frameworks. However, Objective-C does not enforce a universal base class, allowing for the creation of custom root classes.
In contrast, Swift does not have a universal base class, offering more flexibility. Swift classes can stand alone without inheriting from any superclass, or they can inherit from NSObject
when interoperability with Objective-C is required.
Mastering the nuances of root classes in both languages can lead to more effective, maintainable, and scalable code. Whether you’re building new applications in Swift or maintaining legacy Objective-C code, a deep understanding of these concepts will serve as a powerful tool in your development arsenal.