In every programming language, the ability to store and manage data is fundamental. In Swift, the distinction between variables and constants is both clear and essential, influencing how you write and optimize your code.
This blog post explores the concepts of mutability in Swift, comparing them to Objective-C, and discussing the broader implications of mutability in programming.
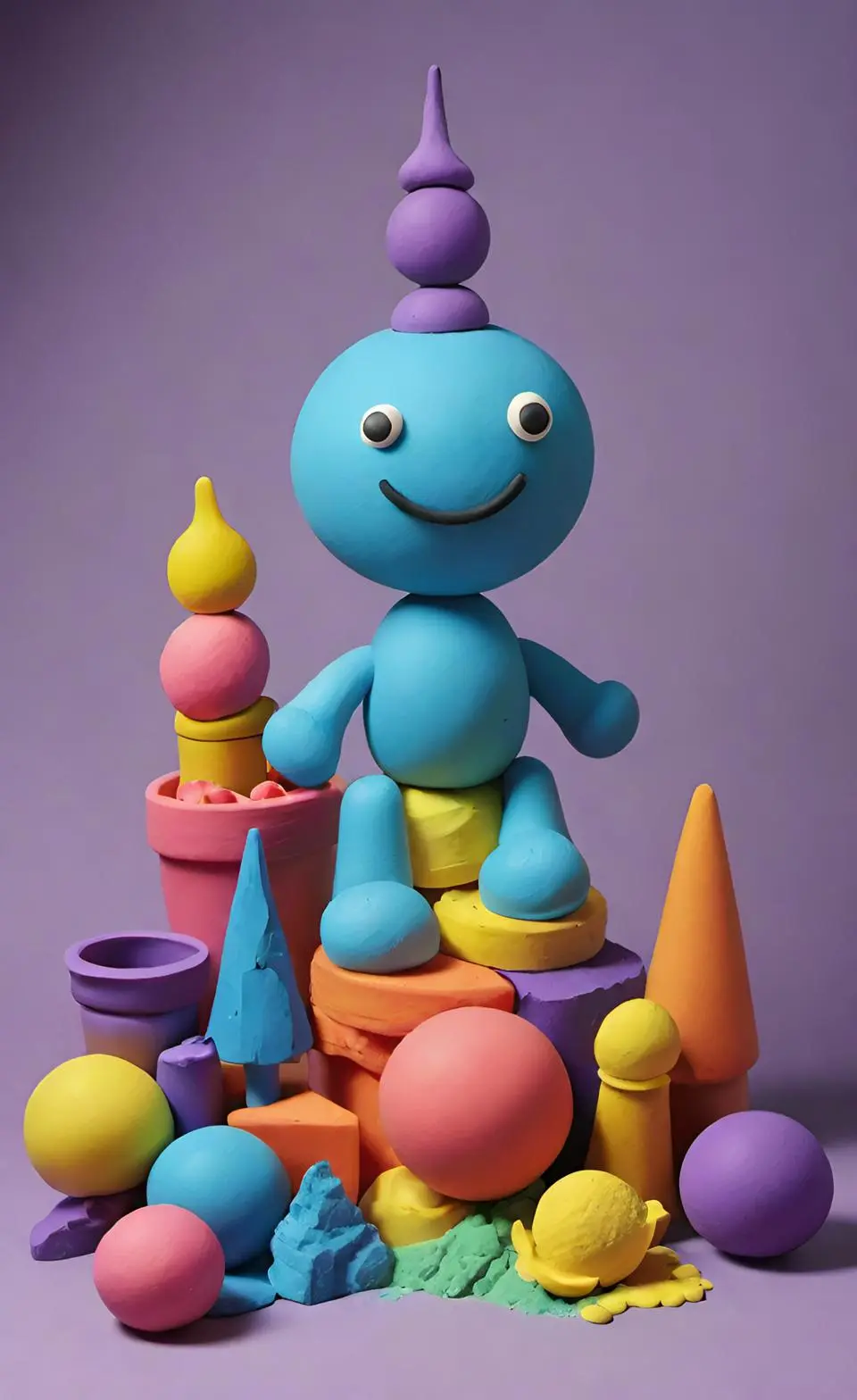
The Power of Mutability in Swift
Swift Variables and Constants: The Basics
In Swift, the way you store data hinges on two keywords: let
for constants and var
for variables. This distinction is not just syntactical but plays a critical role in ensuring the safety, performance, and clarity of your code.
For example, consider a Customer
class in Swift:
class Customer {
let id = 22034
let birthdate = "11/11/2011"
var balance = 109.3
}
Constants cannot change after you run your app, they prevent accidental breakage of a value that should not change.
In this example:
id
andbirthdate
are constants (let
), meaning once they are set, they cannot be changed.balance
is a variable (var
), meaning it can be modified after its initial assignment.
Why Use Constants?
Constants in Swift are more than just a way to store unchangeable data. They offer several advantages:
- Safety: By marking a value as constant, you prevent accidental modifications, which can lead to bugs and unintended behavior in your program.
- Performance: Constants can be optimized by the compiler for better performance. Since their values don’t change, the compiler can make assumptions that lead to faster code execution.
Mutability in Objective-C vs. Swift
Swift’s clear distinction between let
and var
is a marked improvement over Objective-C, where mutability is less explicit. In Objective-C, immutability is often enforced through class hierarchies (e.g., NSString
vs. NSMutableString
) rather than syntax. This can lead to confusion, as the mutability of an object isn’t always immediately apparent from its declaration.
NSString *constName = @"John";
NSMutableString *mutableName = [NSMutableString stringWithString:@"John"];
In the above Objective-C example:
constName
is a constant pointer to an immutableNSString
object.mutableName
is a pointer to a mutableNSMutableString
object.
Swift, by contrast, makes mutability explicit with let
and var
. This clarity reduces the cognitive load on the developer, making the code easier to read, understand, and maintain.
The Broader Implications of Mutability
Mutability is a key concept not just in Swift, but in programming in general. When designing systems, deciding whether an object should be mutable or immutable has far-reaching consequences:
- Concurrency: Immutable objects are naturally thread-safe, as their state cannot change after they are created. This makes them ideal for concurrent programming.
- Design Patterns: Many design patterns, such as the Builder pattern, rely on mutability during object construction and immutability afterward.
- Predictability: Immutable objects lead to more predictable and less error-prone code since their state cannot change unexpectedly.
In Swift, leveraging constants wherever possible can lead to more robust and maintainable code. By defaulting to let
and only using var
when necessary, you reduce the potential for bugs and make your intentions clear to others reading your code.
Conclusion:
Understanding and utilizing Swift’s approach to variables and constants is crucial for writing clean, efficient, and reliable code. By explicitly defining mutability with let
and var
, Swift encourages best practices and helps you avoid common pitfalls that can occur in languages with less clear mutability rules.
Whether you’re transitioning from Objective-C or just starting with Swift, embracing these concepts will elevate the quality of your code and make your applications more robust. Remember, in Swift, clarity is key—so let your constants be constants and your variables be variables, and your code will thank you for it.