As a programmer, you will often need to represent the concept of “nothingness.” In Swift and Objective-C, this idea manifests in various forms: nil
, Nil
, NULL
, and NSNull
.
Each of these serves a distinct purpose and is used in different contexts. Understanding these concepts is fundamental to mastering memory management, type safety, and interoperability between Swift and Objective-C.
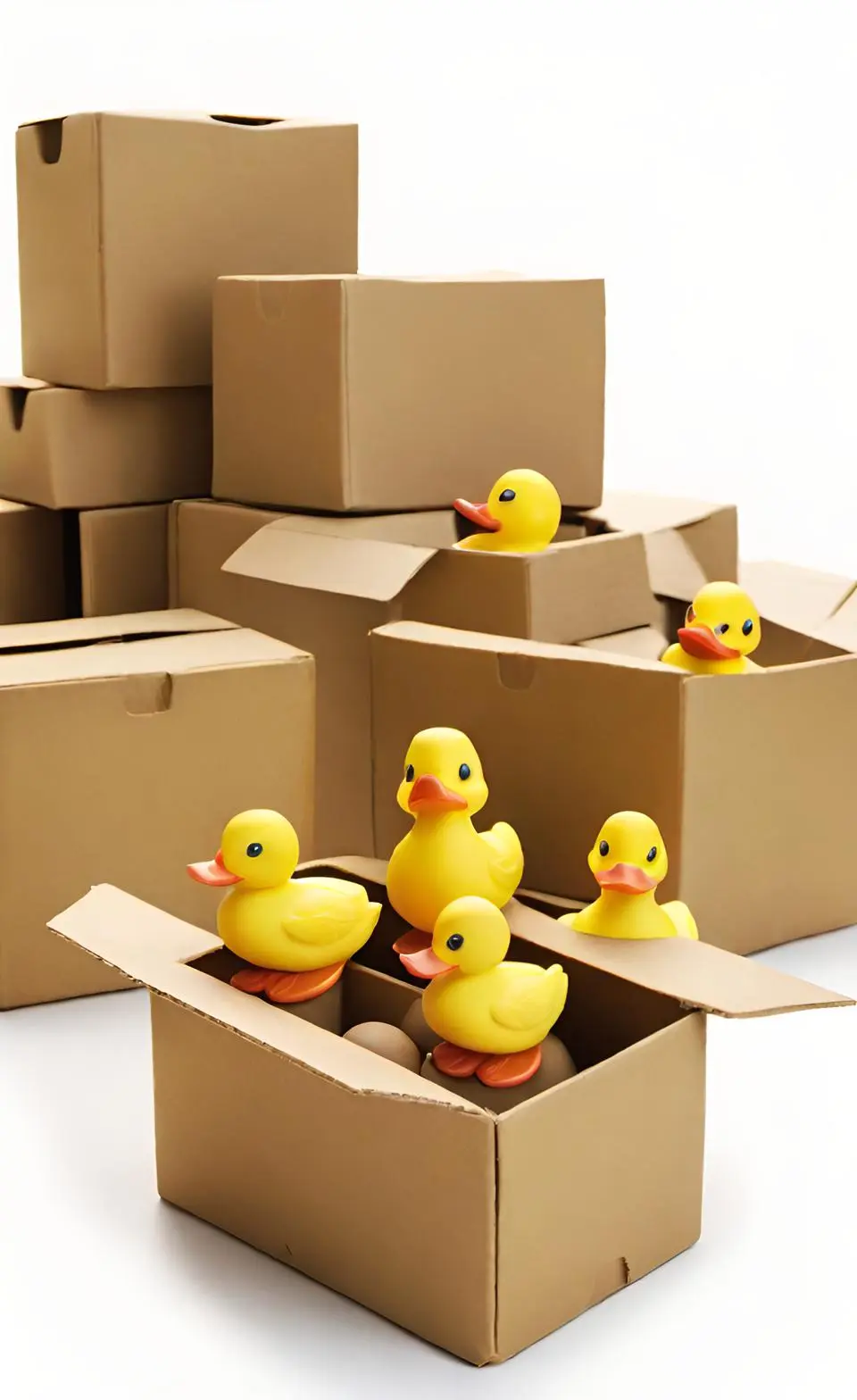
Understanding the Keywords
When dealing with programming languages like Swift and Objective-C, it’s crucial to grasp how they handle the concept of “nothing.” This “nothing” can be expressed in different ways: nil
, Nil
, NULL
, and NSNull
. These terms, while similar in appearance, have distinct meanings and uses.
The Concept of “Null” in Programming
In many programming languages, including C, Objective-C, and Swift, “null” represents the absence of a value or a reference to an object that doesn’t exist. This is a crucial concept because it allows programs to handle cases where data is missing, uninitialized, or optional. However, how “null” is implemented and used can vary between languages and contexts.
NULL
NULL
is a macro in C and Objective-C, typically defined as ((void*)0)
. It represents a null pointer, which is a pointer that doesn’t point to any memory location. In C-based languages, it’s common to use NULL
to indicate that a pointer is not currently pointing to any valid object or memory address. In Objective-C, NULL
is often used for pointers to non-object types, such as structures or primitive data types.
In practice, NULL
is crucial for error handling and conditional logic, allowing developers to check whether a pointer is valid before attempting to use it. For example:
int *ptr = NULL;
if (ptr == NULL) {
// Handle the case where ptr is not pointing to anything
}
nil
In Objective-C, nil
is used to represent a null pointer to an object. It is essentially a way to say “this object does not exist.” Defined as #define nil NULL
, it is functionally equivalent to NULL
but is specifically intended for use with Objective-C objects. When a message is sent to nil
, nothing happens; it returns a value of 0
or nil
, depending on the expected return type. This behavior simplifies error handling in Objective-C, as it avoids crashes that would occur if you attempted to send a message to an uninitialized object.
Here’s an example:
NSString *string = nil;
if (!string) {
// The string is nil, handle accordingly
}
Nil
Nil
is similar to nil
, but it is used specifically for class pointers in Objective-C. When a class variable is Nil
, it indicates that the class does not exist. This distinction is important because Objective-C differentiates between instances of classes (objects) and the classes themselves. For instance, Nil
might be used when checking if a class has been defined or loaded into memory.
NSNull
NSNull
is an Objective-C class that provides a way to represent null values in collection objects such as NSArray
, NSDictionary
, and NSSet
. These collections do not allow nil
values because nil
is used to signify the absence of an object. Instead, [NSNull null]
acts as a placeholder for nil
in these cases.
For example, if you want to store a null value in an array:
NSArray *array = @[[NSNull null]];
In this array, the first (and only) element is [NSNull null]
, representing a null value.
Swift and Objective-C Interoperability
Swift, being a more modern language, handles “nothingness” differently. In Swift, nil
is not a pointer but rather the absence of a value of a certain type. This difference is crucial when working with Swift and Objective-C together, particularly in projects where both languages are used.
Example: Interacting with Objective-C Code in Swift
Consider the following Objective-C code:
// Machine.h
#import <Foundation/Foundation.h>
@interface Machine : NSObject
@property (strong, nonatomic) id serialNumber;
- (void) summary;
@end
// Machine.m
#import <Foundation/Foundation.h>
#import "Machine.h"
@implementation Machine
- (void) summary {
NSLog(@"Initializing Machine");
NSString *label1 = @"label1";
NSString *label2 = nil;
NSString *label3 = [NSNull null];
NSString *label4 = Nil;
NSString *label5 = NULL;
NSLog(@"Label1 = %@",label1);
NSLog(@"Label2 = %@",label2);
NSLog(@"Label3 = %@",label3);
NSLog(@"Label4 = %@",label4);
NSLog(@"Label5 = %@",label5);
}
@end
In Swift, you can interact with this Objective-C code as follows:
let OC_Machine = Machine()
OC_Machine.summary()
OC_Machine.serialNumber = 21
OC_Machine.serialNumber = nil
OC_Machine.serialNumber = NSNull()
//OC_Machine.serialNumber = Nil
//OC_Machine.serialNumber = NULL
print(OC_Machine.serialNumber)
The commented lines will not compile in Swift because Swift does not support the use of Nil
and NULL
in the same way Objective-C does. The output of the above code will be:
Label1 = label1
Label2 = (null)
Label3 = <null>
Label4 = (null)
Label5 = (null)
Optional(<null>)
This example highlights the importance of understanding how each language treats “nothingness” and how these concepts map between Objective-C and Swift.
Summary of Key Points
NULL
is used for non-object pointers in C and Objective-C. It represents a null pointer in a generic sense, applicable to various data types.nil
is used for object pointers in Objective-C, specifically indicating that an object does not exist.Nil
is used for class pointers in Objective-C, representing the absence of a class.NSNull
is a singleton object used to represent null values in collections wherenil
is not allowed.
In Swift, nil
is a fundamental part of the language’s type system and is used to indicate the absence of a value. Unlike Objective-C, where nil
is a pointer, Swift’s nil
is not. Understanding these differences is essential for developers working with both languages, especially in projects that involve interoperability between Swift and Objective-C.
In Objective-C,
nil
is a pointer to a non-existent object. In Swift,nil
is the absence of a value.
NULL
andnil
are equal to each other in Objective-C, butnil
is used specifically for object pointers, whileNULL
is for generic pointers.[NSNull null]
is an object meant to representnil
in contexts wherenil
is not allowed, such as in collections.
By mastering these concepts, you’ll be better equipped to handle memory management and interoperability challenges in both Objective-C and Swift.