In Swift, optionals play a crucial role in managing situations where a value might be absent. Unlike Objective-C, where nil
can be assigned to any object pointer without much regard for safety, Swift’s optionals provide a more structured and secure way to represent missing data.
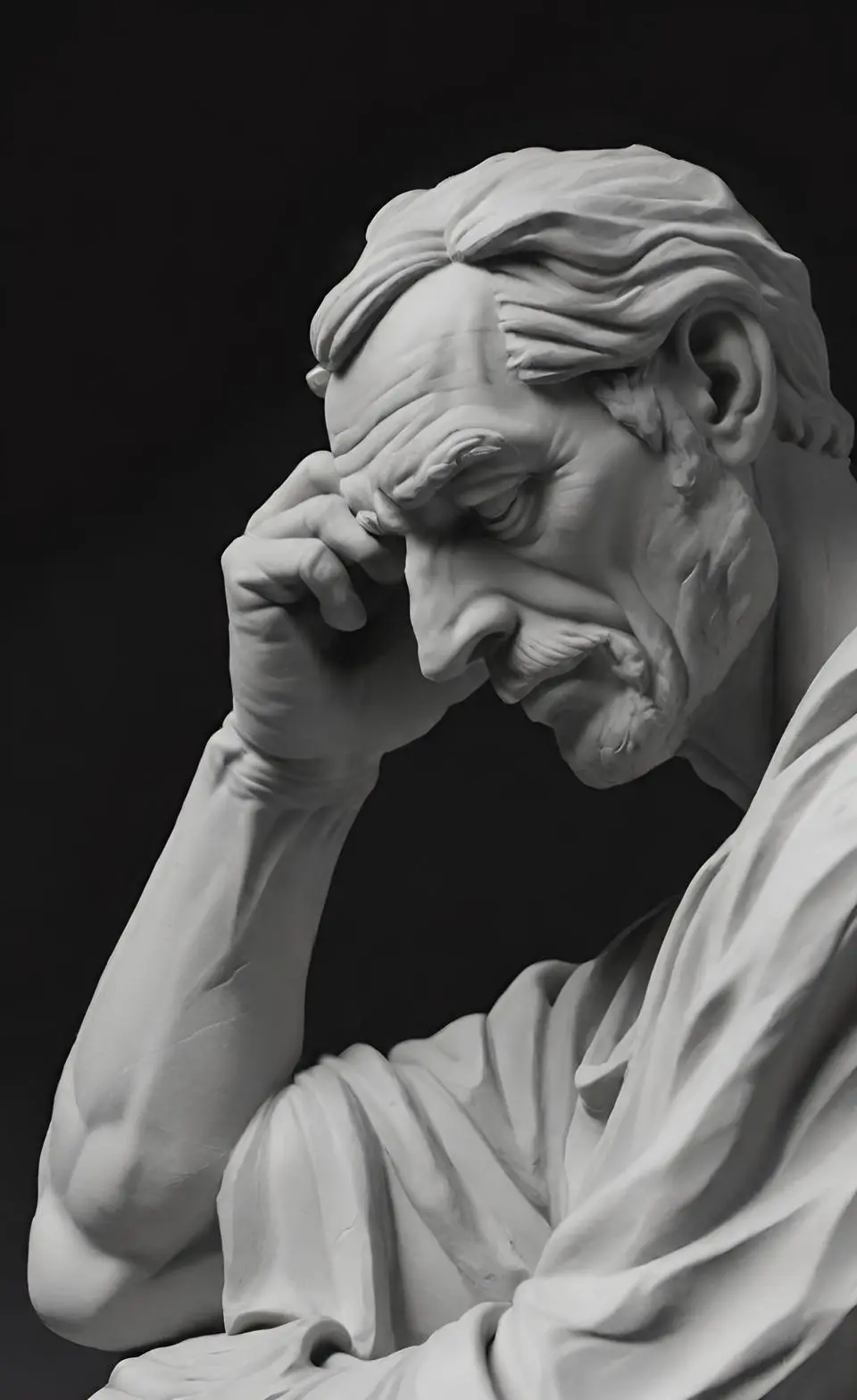
What are Optionals?
Optionals in Swift are types that can hold either a value or no value at all. In essence, they represent the possibility of absence, which helps in safely dealing with missing or unavailable data. You declare an optional by appending a ?
after the type of the variable:
var name: String?
This means that the name
variable can either hold a String
or be nil
. Without Swift’s optionals, we would need to rely on cumbersome manual checks or risk unhandled nil
values, which could cause crashes.
Optionals are Enums
At its core, an optional in Swift is actually an enumeration with two cases:
enum Optional<Wrapped> {
case none
case some(Wrapped)
}
This means an optional either holds a value (some
) or does not hold a value (none
). The fact that it’s an enum gives Swift the ability to represent the absence of data in a more structured way compared to traditional nil
checks found in other languages like Objective-C.
Why Optionals?
Optionals are a powerful feature of Swift for several reasons:
- Type Safety: Swift forces developers to think explicitly about the absence of a value. A variable cannot hold
nil
unless it’s an optional. - Avoid Crashes: Accessing
nil
values in Swift is managed more gracefully, reducing the chances of runtime crashes due to unintentional use of nil objects. - Clear Intentions: Optionals make your intentions clear in code. When you use an optional, it signals to others (and to yourself) that the value may or may not be present.
Unwrapping Optionals
Since optionals can either have a value or be nil
, you cannot access their values directly. You must first unwrap the optional to access the underlying data. Swift offers several ways to do this safely:
1. Forced Unwrapping
If you are absolutely certain that an optional contains a value, you can force unwrap it by adding a !
after the optional variable:
let unwrappedName: String = name!
However, if the optional is nil
and you attempt to force unwrap it, your program will crash. This is why it’s important to use forced unwrapping cautiously.
2. Optional Binding
A safer and more recommended approach is to use optional binding with if let
or guard let
. This allows you to unwrap the optional only if it contains a value:
if let unwrappedName = name {
print("The name is \(unwrappedName).")
} else {
print("The name is nil.")
}
Optional binding ensures that you only work with a value if it’s available, preventing unnecessary crashes.
3. Nil Coalescing
Sometimes, you want to provide a default value if the optional is nil
. You can do this with the nil coalescing operator (??
):
let displayedName = name ?? "Anonymous"
In this case, if name
is nil
, the string “Anonymous” will be used instead.
Optional Chaining
Swift allows you to safely query and call properties, methods, and subscripts on optionals that might currently be nil
. This is called optional chaining. Instead of checking if the optional is nil
before accessing its properties, you can append a ?
after the optional and safely proceed:
let uppercaseName = name?.uppercased()
Here, if name
is nil
, uppercaseName
will simply be nil
rather than causing a crash.
Implicitly Unwrapped Optionals
In some cases, you might want to declare an optional that will always have a value after being set once. In such cases, you can use implicitly unwrapped optionals:
var email: String!
This tells the compiler that email
may be nil
initially, but once it is set, it can be used without the need for unwrapping. This is useful in situations like initialization, where a value is guaranteed to exist after a certain point.
Example of Usage
Let’s look at a real-world example where optionals are useful. Consider an app that retrieves user information from a server, which may or may not provide a user’s nickname. Without optionals, handling missing values would be error-prone. Here’s how you might handle this in Swift:
struct User {
var name: String
var nickname: String?
}
let user = User(name: "Alice", nickname: nil)
if let nickname = user.nickname {
print("Nickname: \(nickname)")
} else {
print("No nickname available.")
}
Avoiding Common Pitfalls
Although optionals provide many benefits, it’s essential to use them wisely. Here are a few tips:
- Avoid overusing implicitly unwrapped optionals. These can lead to unexpected crashes if not managed properly.
- Be mindful of forced unwrapping (
!
). Only force unwrap optionals when you are absolutely certain that they hold a value. - Use optional binding wherever possible to safely unwrap values and handle cases where data might be missing.
Conclusion
Swift’s optionals are a game-changer in ensuring safer and more robust code. By enforcing explicit handling of nil
values, optionals help you avoid many common bugs and crashes. Whether you’re working on a small project or a large iOS application, understanding and using optionals effectively is key to writing reliable Swift code.
By mastering optionals, you’ll be able to tackle Swift’s type-safe approach to handling the absence of data confidently. Happy coding!