Swift provides a rich array of built-in data types that are designed to accommodate various kinds of data, each serving a specific purpose in programming. Understanding these data types is crucial for developers aiming to write efficient, reliable, and bug-free code.
By grasping the nuances of each type, programmers can optimize memory usage, ensure type safety, and enhance the overall performance of their applications. This foundational knowledge not only aids in effective data manipulation but also fosters better coding practices, leading to cleaner and more maintainable code.
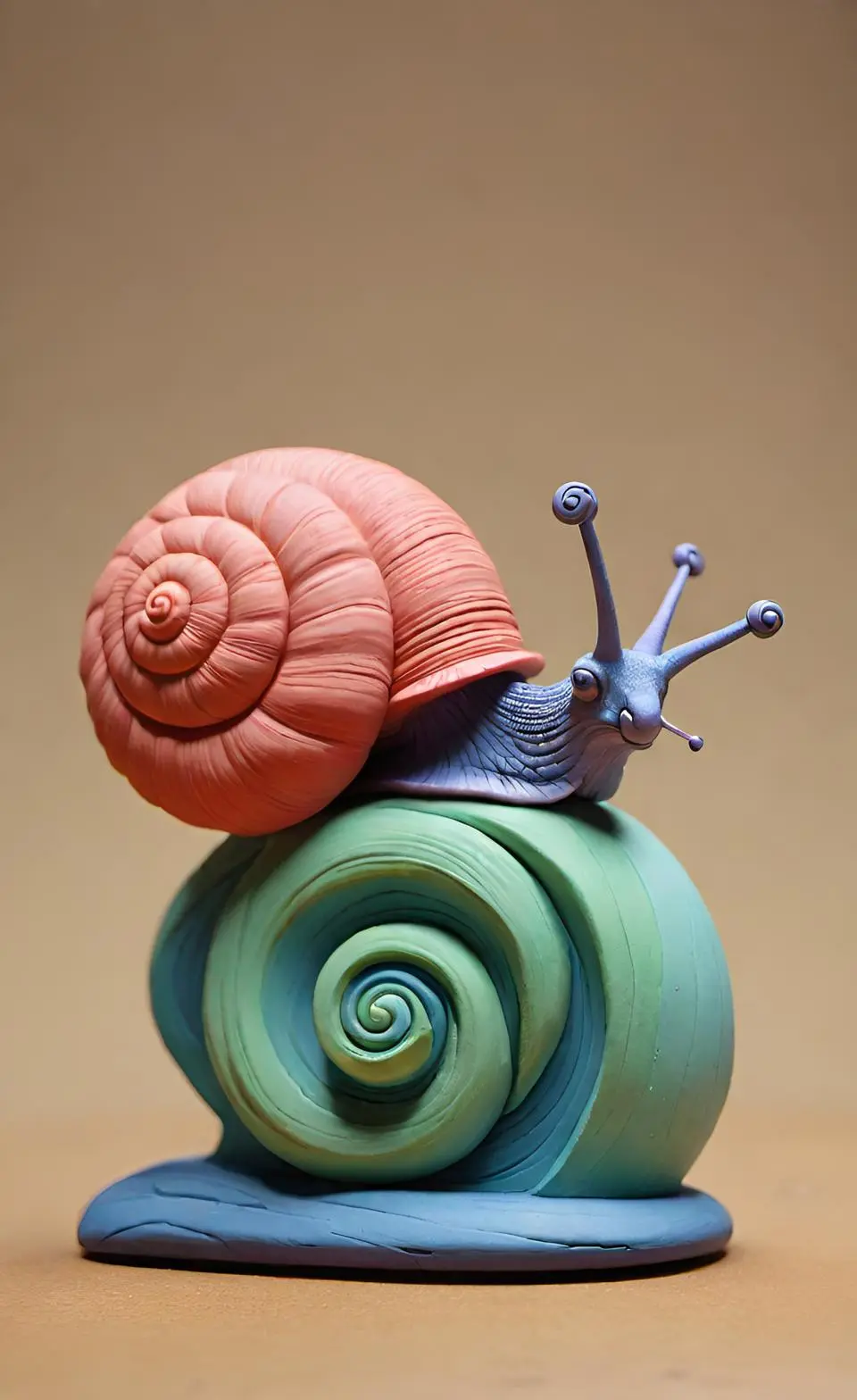
Swift’s Basic Data Types
Below is an overview of the primary data types available in Swift:
Type | Description |
---|---|
Character |
Represents a single 16-bit Unicode character, like "a" or "/" . |
String |
Represents a sequence of characters, used for textual data like "Hello" . |
Float |
A 32-bit floating-point number, useful for representing fractional values. |
Double |
A 64-bit floating-point number, offering double the precision of a Float . |
Bool |
Represents a Boolean value, which can be either true or false . |
Tuples |
Groups multiple values into a single compound value, e.g., (1, "Hello") . |
Int |
Represents a signed integer (whole number). On 32-bit platforms, it is Int32 ; on 64-bit platforms, it is Int64 . |
UInt |
Represents an unsigned integer (whole number). On 32-bit platforms, it is UInt32 ; on 64-bit platforms, it is UInt64 . |
Int8 |
A signed 8-bit integer, capable of storing values from -128 to 127 . |
Int32 |
A signed 32-bit integer, common in many programming tasks. |
Int64 |
A signed 64-bit integer, offering a wider range of values. |
UInt8 |
An unsigned 8-bit integer, with a range of 0 to 255 . |
UInt32 |
An unsigned 32-bit integer, often used in systems programming. |
UInt64 |
An unsigned 64-bit integer, useful for representing large positive integers. |
Device-Specific Integer Sizes
On 32-bit devices, the Int
type has the size of Int32
, while on 64-bit devices, it has the size of Int64
. The same principle applies to UInt
, where its size depends on the architecture of the device.
Type Inference in Swift
One of Swift’s powerful features is type inference, which allows the compiler to automatically determine the type of a variable based on the value assigned to it. This can significantly reduce the amount of boilerplate code and make the code more readable.
Example of Type Inference
Consider the following example, where the type of the variable level
is inferred by Swift:
var level = 12
Here, Swift automatically infers that level
is of type Int
because 12
is an integer. The compiler’s ability to infer types simplifies the code, especially in cases where the type is obvious from the context.
Explicit Type Annotation
While type inference is a valuable feature, there are scenarios where explicitly specifying the type is beneficial or even necessary. For instance, when dealing with complex data structures or when the inferred type may not be immediately clear to someone reading your code, explicit type annotations enhance readability and maintainability.
var level: Int = 17
In this case, even though the type could be inferred, declaring it explicitly as Int
improves clarity, particularly in larger codebases where understanding the intent behind each variable is crucial.
The Impact of Type Inference on Performance
Type inference in Swift, while primarily aimed at improving code readability and reducing boilerplate, also has implications for performance. The Swift compiler is designed to be highly efficient, and type inference plays a role in this efficiency. However, understanding when and how type inference affects performance can help developers make informed decisions in critical parts of their code.
Compilation Performance
One area where type inference can impact performance is during the compilation process. When Swift infers types, the compiler has to analyze the code more extensively to deduce the correct types, which can slightly increase compilation times, especially in large codebases with complex type relationships. While this overhead is generally minimal, it can become noticeable in very large projects or in code that makes heavy use of generic types and protocols.
Runtime Performance
At runtime, Swift’s type inference has little to no impact on the performance of the compiled code. The inferred types are determined at compile time, meaning that by the time your application is running, the types are already fixed and optimized by the compiler. This ensures that the performance of your application is consistent, regardless of whether types were inferred or explicitly declared.
Best Practices for Performance
To optimize both compile-time and runtime performance, consider the following best practices:
- Explicit Type Annotations in Complex Expressions: In scenarios where complex expressions are used, explicitly annotating types can help the compiler optimize the code more effectively, reducing compilation time.
- Avoid Overuse of Type Inference in Critical Paths: In performance-critical code, such as in tight loops or algorithms, explicit types can sometimes lead to more predictable and optimized compiled code.
- Balance Readability and Performance: While type inference makes code more concise, always balance this with the need for clarity and performance. In complex or critical sections of your code, it might be beneficial to favor explicit type declarations.
By being mindful of when and where to rely on type inference, you can write Swift code that is both efficient and maintainable, ensuring that your applications perform optimally without sacrificing code quality.
Unsafe Types in Swift
In addition to the safe and type-safe data types that Swift provides, there are also “unsafe” types that allow developers to work with memory directly. These types are primarily used in scenarios where performance is critical, or when interfacing with lower-level APIs, such as C libraries. While they offer greater flexibility and control, they come with increased risks, as they bypass Swift’s safety features.
1. UnsafePointer
UnsafePointer
is a type that provides a pointer to a memory location. It allows you to read values from memory without the safety guarantees that Swift typically enforces. Here’s an example of how to use UnsafePointer
:
let numbers: [Int] = [1, 2, 3, 4, 5]
let pointer = UnsafePointer(numbers)
print(pointer[0]) // Access the first element
2. UnsafeMutablePointer
UnsafeMutablePointer
allows both reading and writing of values at a specific memory location. This is useful when you need to modify the contents of an array or a buffer directly:
var mutableNumbers: [Int] = [1, 2, 3, 4, 5]
let mutablePointer = UnsafeMutablePointer(&mutableNumbers)
mutablePointer[0] = 10 // Change the first element to 10
print(mutableNumbers) // Output: [10, 2, 3, 4, 5]
3. UnsafeRawPointer
UnsafeRawPointer is a pointer that points to raw memory without any type information. This can be useful for low-level operations, such as when working with binary data or interfacing with C APIs:
var mutableNumbers: [Int] = [1, 2, 3, 4, 5]
let mutablePointer = UnsafeMutablePointer(&mutableNumbers)
let rawPointer = UnsafeRawPointer(mutablePointer)
let intPointer = rawPointer.assumingMemoryBound(to: Int.self)
print(intPointer[0]) // Access the first element as an Int
CGFloat: A Special Case
A noteworthy data type in Swift is CGFloat
, which is particularly relevant when dealing with graphical calculations in iOS and macOS applications. CGFloat
can represent either a 32-bit or 64-bit floating-point number, depending on the device’s CPU architecture. This makes it a versatile choice for applications that require precise and scalable graphical calculations, such as in drawing operations or animations.
Understanding Swift’s data types and effectively using type inference can lead to more concise, readable, and safer code. By mastering these fundamentals, you can write Swift code that is not only efficient but also easy to maintain and extend as your projects grow.