Swift is Apple’s modern, open-source programming language, introduced at Apple’s 2014 Worldwide Developers Conference (WWDC). Designed to be the successor to Objective-C, Swift is used for developing applications across all of Apple’s platforms, including iOS, macOS, watchOS, and tvOS.
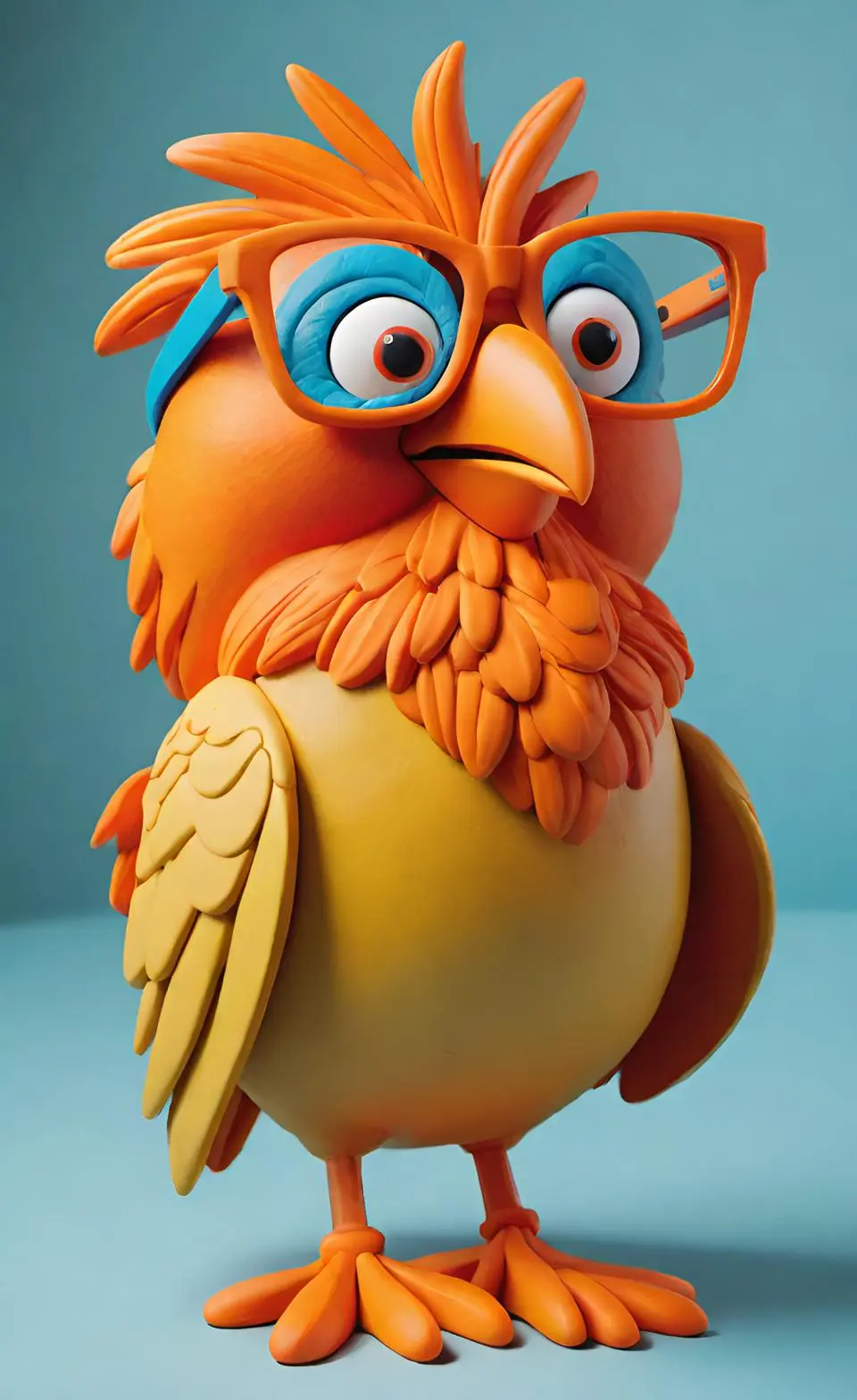
It primarily works with Apple’s Cocoa
and Cocoa Touch
frameworks. Since its introduction, Swift has rapidly become one of the fastest-growing programming languages. As an open-source language licensed under the Apache 2.0 license, Swift has extended beyond Apple’s ecosystem, finding use in other areas such as backend server development with frameworks like Vapor
.
Key Features of Swift
Swift was designed with several goals in mind: to be concise, expressive, fast, and less prone to errors (safer) than its predecessor, Objective-C. It introduces modern language features that make it easier to write reliable and maintainable code.
The Architect of Swift
Chris Lattner, the principal designer of Swift, was a key figure at Apple Inc., where he served as the Director of the Developer Tools department. In this role, he led the teams behind Xcode, Instruments, and the compiler technologies. Lattner is also the main author of LLVM (Low-Level Virtual Machine), a compiler infrastructure, and Clang, a compiler front end that replaces the GNU Compiler Collection and is designed to work atop LLVM.
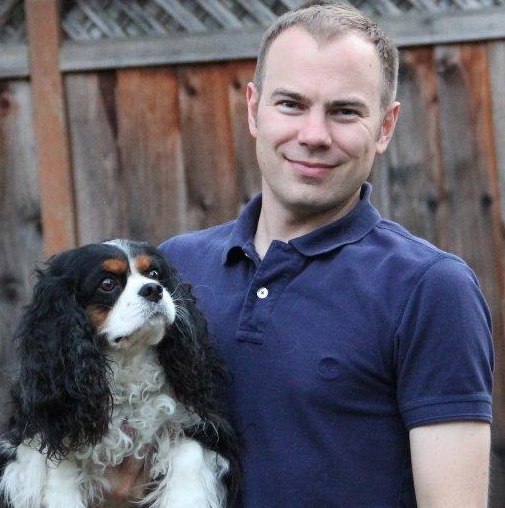
Chris Lattner (Twitter @clattner\_llvm)
Swift’s Versatility and Ease of Learning
To facilitate onboarding for newcomers, Swift can be used in various environments. One of the most accessible is the Swift Playgrounds, an interactive environment in Xcode, or through a web-based REPL (Read-Eval-Print Loop) like this one. A REPL provides a command-line interface that allows developers to experiment with Swift in an interpreted-like environment, making it easy to learn and test new code snippets quickly.
Swift: A General-Purpose, Multi-Paradigm Language
Swift is a type-safe, general-purpose, and multi-paradigm language. It supports a wide range of programming paradigms, including object-oriented, functional, and generic programming. This flexibility allows developers to use the most suitable style for a given problem, recognizing that no single paradigm is perfect for all use cases. Swift provides its own versions of common C and Objective-C types, alongside powerful data structures such as Arrays, Sets, and Dictionaries. Moreover, it includes unique types like tuples, which enable the passing of grouped values—a feature not available in Objective-C.
Security & Swift/Objective-C
While both Swift and Objective-C can be used to develop secure iOS applications, Swift offers several security advantages due to its modern language design:
-
Type Safety and Optionals: Swift enforces strict type safety, reducing the risk of type-related errors that can lead to security vulnerabilities. Its use of optionals requires developers to handle
nil
values explicitly, minimizing the risk of runtime crashes or unexpected behavior, unlike Objective-C wherenil
values can silently fail. -
Memory Management: Both Swift and Objective-C use Automatic Reference Counting (ARC) for memory management, but Swift’s syntax and stricter rules make it easier to avoid common pitfalls like retain cycles and dangling pointers. Objective-C’s more permissive nature increases the potential for memory-related errors.
-
Bounds Checking: Swift automatically performs bounds checking on arrays and collections, which helps prevent buffer overflow vulnerabilities. In Objective-C, developers need to manually ensure that data access stays within valid bounds, increasing the risk of out-of-bounds errors.
-
Immutable by Default: Swift encourages safer code by making constants (
let
) immutable by default. Objective-C requires the use of specific class types for immutability (e.g.,NSString
vs.NSMutableString
), making the codebase more prone to unintended modifications if not carefully managed. -
Reduced Use of Unsafe Code: Swift avoids certain low-level operations, such as direct memory manipulation with pointers, that are more prevalent in Objective-C. This reduces the attack surface for exploits like buffer overflows and memory corruption.
-
Enhanced Privacy and Security Practices: Swift’s language features align well with modern privacy regulations (e.g., GDPR, CCPA), supporting secure coding practices more intuitively and allowing developers to implement security measures more easily compared to Objective-C.
These features make Swift a more secure choice for developing iOS applications, as it reduces common programming errors and provides built-in safeguards against many vulnerabilities.
Swift Projects and Ecosystem
The Swift programming language is made up of several key components, each of which contributes to its versatility and power. According to Swift.org, these include:
- The Swift compiler, a command-line tool for compiling Swift code.
- The Standard Library, which provides essential functionality and is bundled with the language.
- Core Libraries that offer higher-level capabilities.
- The LLDB Debugger, which includes the Swift REPL, enabling debugging and interactive coding.
- The Swift Package Manager, a tool for managing and building Swift projects.
- Xcode Playground Support, which enables the use of playgrounds in Xcode for interactive coding.
Swift continues to evolve, driven by contributions from both Apple and the open-source community. Its combination of speed, safety, and expressiveness makes it a strong choice for developers looking to build modern applications across multiple platforms.